Optimizing Performance in .NET Core: Tips and Techniques
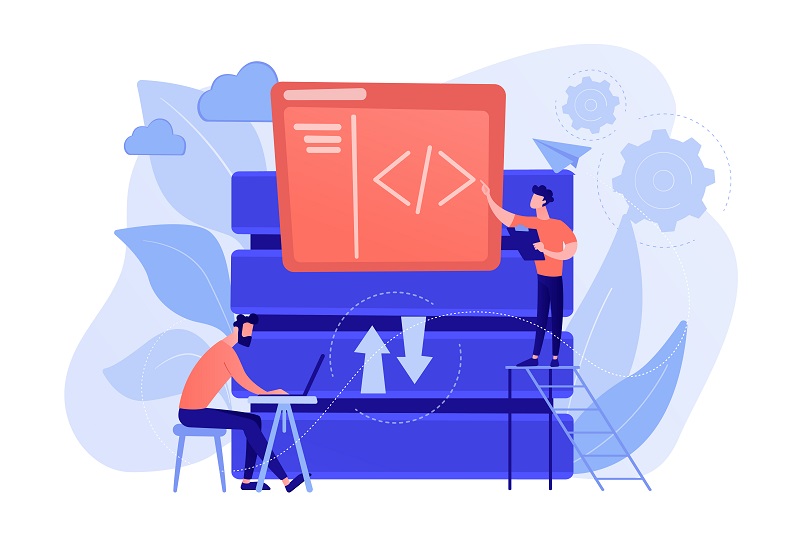
With the ever-increasing complexity of applications and the need for scalability, it is crucial to leverage the full potential of .NET Core and its performance optimization capabilities. From efficient coding practices and memory management techniques to utilizing caching mechanisms and optimizing database queries, we will cover a wide range of strategies. This will boost the speed and responsiveness of your applications.
As a developer, you understand the importance of delivering fast and efficient software that meets the demands of modern users. In this blog, we will delve into a variety of tips and techniques to help you optimize the performance of your .NET Core applications and deliver a seamless user experience.
Whether you’re a seasoned .NET Core developer or just starting with the framework, these tips and techniques will provide you with valuable insights and practical guidance to fine-tune your application’s performance. So, let’s embark on this journey of optimizing performance in .NET Core and unlock the full potential of your applications.
Introduction – Need for .NET core performance optimization
In software development, performance optimization is essential, and .NET Core offers a number of tools and methods to improve the speed of applications. Here are some justifications for why .NET Core performance optimization is crucial:
– Scalability –
Applications can scale efficiently and withstand greater workloads thanks to .NET core performance optimization. They can accept more users and process more requests without sacrificing speed by reducing resource utilization and enhancing efficiency.
– Speed –
Performance optimization ensures that .NET Core-based apps react rapidly to user input, enhancing the user experience as a whole. Increased user involvement and satisfaction are the results of quicker response times.
– Cost-effectiveness –
Applications that have been properly optimized use fewer resources, which reduces the cost of infrastructure and hosting. Organizations may effectively use their resources and improve cost efficiency by lowering memory consumption, CPU cycles, and network traffic. Thus .NET core performance optimization is a win-win situation.
– Competitiveness –
Performance is crucial for user retention and getting a competitive edge in the fast-paced industry of today. Applications that have been optimized provide consumers with a quicker and more effective experience while surpassing those of rivals.
- The cloud is a good environment for .NET Core installations. Applications may utilize the flexibility and scalability of the cloud to their full potential with the help of performance optimization. Organizations may maximize the advantages of cloud computing by streamlining resource utilization and speeding up response times. .NET core performance optimization is unavoidable.
Developers may use methods like code profiling, caching, asynchronous programming, etc to optimize speed in .NET Core. Let’s discover the ways and techniques which lead to the best .NET core throughput.
Contrivances to achieve top-notch .NET core optimization
Here are some ways that can boost the core-functioning outcome.
- Program asynchronously – Performance may be enhanced by using async/await and tasks for asynchronous programming, which allows several operations to run simultaneously. Applications may effectively use system resources and retain responsiveness by minimizing blocking calls, which helps for .NET Core performance optimization.
- Reduce the pressure on garbage collection (GC) – Overhead can be created by the .NET Core garbage collector. Reducing pointless object allocations, using object pooling, and optimizing memory use are actions that must be taken in order to optimize .NET Core performance optimization. Performance may be greatly increased by reusing existing objects rather than making new ones.
- Improve Database Access – Efficiently accessing databases is crucial for achieving .NET Core performance optimization. Techniques like batch processing, caching frequently accessed data, optimizing queries, and using asynchronous database operations can enhance application performance. Utilizing an ORM (Object-Relational Mapping) tool like Entity Framework Core with query optimizations can also contribute to .NET Core performance optimization.
- Set Response Compression to “On” – Response compression is a valuable strategy for achieving .NET Core performance optimization. Response times are accelerated when response compression is enabled in .NET Core because it decreases the amount of data that has to be transmitted over the network. Performance can be enhanced by applying Gzip or Brotli compression to static files and API replies, especially when dealing with big payloads.
- Use AspNetCore.ResponseCaching – By caching HTTP replies, the built-in response caching middleware in ASP.NET Core can enhance performance for online applications. As a consequence, fewer requests require content regeneration, which speeds up response times and improves the .NET Core performance optimization. Response caching enables the application to provide directly cached responses to the client without having to restart the full request pipeline, resulting in considerable speed benefits.
- Performance profiling should be used – Performance bottlenecks in .NET Core apps may be found using profiling tools like dotMemory, dotTrace, or Visual Studio Profiler. Developers may identify areas for optimization and make informed performance enhancements by looking at memory use, CPU utilization, and execution pathways.
- Take into account containerization and microservices – Better scalability and performance optimization are made possible by splitting up large, monolithic programs into more manageable, independently deployable microservices. By simplifying deployment and enabling effective resource utilization through containerization, performance and scalability are increased. .NET core performance optimization should be bypassed as something of low importance.
- Make use of JIT Optimisation – Code execution at runtime may be optimized thanks to the Just-In-Time (JIT) compiler in .NET Core. This enhances the .NET core performance optimization, by making sure that the code is JIT-friendly by minimizing pointless boxing/unboxing, minimizing the use of reflection, and using value types.
- Benchmark and do load testing – Benchmarking and load testing are useful for detecting performance constraints and bottlenecks for .NET core performance optimization. With the help of tools like Apache JMeter or wrk, developers may fine-tune performance optimizations based on actual use cases by simulating heavy user loads and stress-testing programs.
- Efficiently Perform Asynchronous I/O Operations: When it comes to I/O operations, performing them asynchronously is crucial to ensure minimal impact on other processes. I/O operations typically involve tasks such as uploading or retrieving files, including image or file uploading. However, attempting to execute these operations synchronously can lead to blocking the main thread and halting other background processes until the I/O task is completed. To maintain optimal performance, it is recommended to utilize asynchronous execution for I/O operations. Thankfully, there are numerous asynchronous methods available for handling I/O tasks, such as ReadAsync, WriteAsync, and FlushAsync.
- Boost Application Performance with Effective Caching: One effective strategy to enhance the performance of an application is to reduce the number of requests made to the server. However, this does not imply eliminating server calls altogether. Instead, implementing caching allows us to store and retrieve responses, thereby reducing the need for repetitive server requests.
Caching involves making an initial request to the server and storing the response for future use within a specified timeframe. Subsequent requests for the same data can then be served using the stored information, eliminating the need for additional server calls. Implementing caching is considered a best practice as it significantly improves application performance. There are various caching options available in Professional asp.net development services, such as in-memory caching, response caching, and distributed caching. Each option offers different benefits and can be applied at various levels, including client-side caching, server-side caching, or a combination of both. By leveraging caching effectively, we can minimize server load, reduce response times, and optimize the overall performance of the application.
- Enhancing Performance with Entity Framework Core Query Optimization- Entity Framework Core (EF Core) is a powerful ORM tool that simplifies database interactions for .NET developers. However, the performance of an application heavily relies on the efficiency of its data access logic. By optimizing the code that interacts with the database using EF Core, we can significantly improve application performance. Here are some techniques to achieve this:
-
- Use No Tracking: When fetching data for read-only purposes, utilize the No Tracking feature in EF Core. This improves performance by eliminating the overhead associated with change tracking.
-
- Filter Data at the Database Side: Instead of retrieving the entire dataset and filtering it locally, leverage EF Core functions like Where and Select to filter data at the database level. This reduces the amount of data transferred between the database and the application.
-
- Implement Pagination: Retrieve only the required number of records using the Take and Skip methods. This is particularly useful for implementing paging functionality, where you can efficiently navigate through large datasets.
-
- By applying these optimization techniques, you can enhance the performance of your application, ensuring efficient data access and improved responsiveness.
Conclusion
In summary, optimizing the performance of .NET Core is vital for creating high-performing applications. By implementing various techniques such as asynchronous programming, reducing garbage collection load, optimizing database access, enabling response compression, and implementing caching systems, developers can significantly enhance the speed and efficiency of .NET Core apps.
The robust and flexible nature of the .NET Core framework allows for the development of cross-platform applications, and performance optimization ensures these apps deliver fast response times, efficient resource utilization, and enhanced scalability. By prioritizing performance optimization, businesses can offer a seamless and responsive user experience, leading to increased customer satisfaction, engagement, and a competitive edge in the market.