Advanced UI Development in Flutter: Tips and Tricks
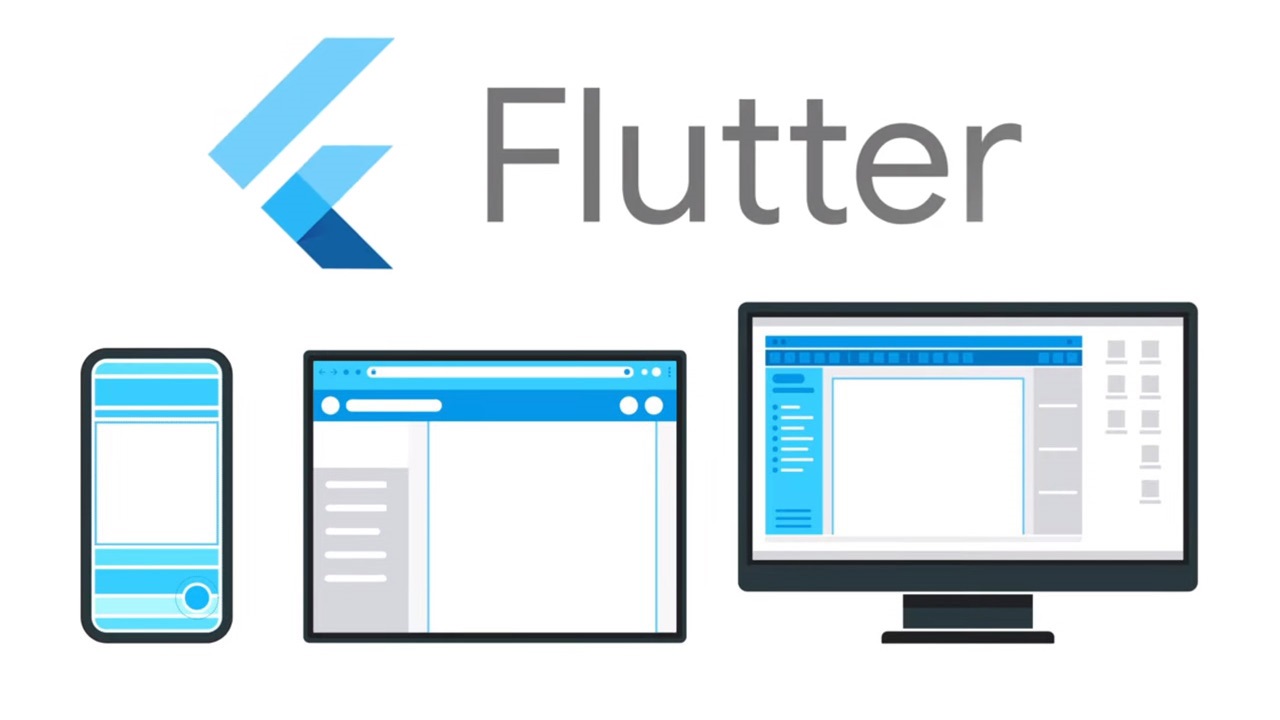
If you are an intermediate Flutter developer, you may be familiar with some of the basics of UI development in Flutter. You’ve learned how to create layouts with basic widgets and how to respond to user input with buttons and text fields. But there’s so much more that you can do with Flutter! This guide will show you how to create advanced user interfaces in Flutter, from the simplest of lists to complex forms and elaborate layouts that rival native applications in design and functionality. If you want to take your UI development skills to the next level, this guide has what you need!
Adding an Animated Loading Widget
In this section, we will develop a widget that displays an animated loading animation. The demo app shows how to create a TextFormatter subclass for the percentage complete message, create an AnimationController and load it with the default Animation using its loadFromKinematics() method. This is needed because loading animations usually involve some kind of non-trivial physics simulation. In order to smoothly animate our TextView widget, we need to use an animation controller that tracks values over time.
Encapsulating Forms Into Modules
When working with forms, one of the first things to do is to create a Material Design button (so that the user knows that they are interacting with a form). This can be done by following these steps:
1. Add a Grid item to your layout. This will give you room for 2 columns and 3 rows.
2. Set up two separate modules – one for the row above the grid and one for the row below it.
3. Set up five columns of varying heights, each starting with the Row widget 1px high so that there is some padding between them.
Implementing Context Menus
Let’s go over how to create context menus! You can do this by first implementing a `ContextMenuController` widget. This will be used as the container for any context menu content that you want to show. Next, initialize the controller with an existing Widget which is where we want the context menu to be shown when pressed. In our example, we use an Image widget. To specify which type of gesture should trigger the appearance of the controller, we have to assign it either one of the FLUTTER_GESTURE_WITHIN_RECT or FLUTTER_GESTURE_WITHIN_FRAME gestures.
Using ValueAnimators to Create Animations
One of the best ways to create animations with Flutter is to use ValueAnimators. These are easy ways to gradually transition between different values on a Widget or StatefulWidget (object).
The animation has a start value, an end value, duration, and timing function as parameters. The transitions can either be linear or easing between two points. Easing can also be applied if there are two curves used for the animation.
Dealing with Nested Screens
A common use of nested screens is to progressively reveal information. Consider the case where we want to start with a list of items. We may be tempted to just instantiate a ListView widget for this, but if we have multiple levels of nesting then it would be preferable for our ListView widgets to be embedded within other widgets that could then act as containers for those lists. To do this, we can define a layout manager on each nested ListView widget which will ensure they are laid out appropriately and don’t end up overlapping.
Add Panels, Not Slots, To Your Widgets
In many cases, you’ll want to have multiple widgets which are positioned on the screen at once. The typical approach is to set up each widget inside a container widget, and then add it to the screen with a Row, Column or another layout constructor. Unfortunately, this approach can lead to overlapping widgets if you resize the screen since changing the size will change the order of the children of the parent widget. In order to avoid this problem, use AddPanel() instead of a slot(). This way, adding or removing panels does not affect their children because they’re removed from all layouts automatically if necessary; their position doesn’t depend on how their parent’s size changes.
Focus On Errors, Not Successes
When building a user interface, it’s natural to focus on what looks good, not what doesn’t. But just because your app is visually attractive doesn’t mean it’s going to be the best for your users. Errors like spacing issues, and missing or duplicated items are often overlooked until they impact the user experience – which is never fun for anyone. So don’t forget that advanced UI design also includes making sure everything on the screen works as intended without glitches or bugs!
Use Custom Types for All Circular Dependencies Between Widgets
The larger the application, the more interconnectedness we will have between our widgets. Sometimes it is difficult to keep track of circular dependencies like this when programming our app. Luckily, with flutter, there are tools to help us use custom types for all circular dependencies between widgets! Doing this makes debugging easier because we can add specific breakpoints for each custom type, allowing us to step through our code and pinpoint which dependency caused a crash.
Conclusion –
Focusing on what you do best, rather than becoming a jack of all trades, is an essential principle for a successful developer. Invest your time developing strong skills in the languages you want to excel at and hire a Flutter development company to handle the rest!